Turn Signals
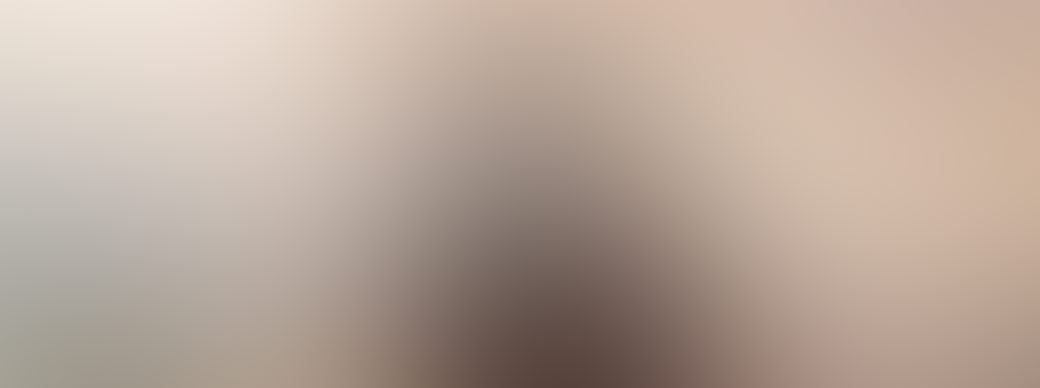
In order to set up the turn signals on the RaspberryPi, a method of wireless communications needed to be set up between the Jetson TX2, and the Raspberry Pi 3. We decided to use Bluetooth for its simplicity. The Jetson TX2 was set up as a server, sending data to the Raspberry Pi, which was set up as a client. The example code for the client.c and server.c was sourced from https://people.csail.mit.edu/albert/bluez-intro/x502.html. The only things that were changed were the hard coded addresses, and the data that is sent to the Raspberry. For now, the program only sends simple signals to the Raspberry Pi, and the Raspberry Pi will output text based on the signal sent.
First the following line was needed to install bluetooth dev capabilities on the Jetson:
sudo apt-get install libbluetooth-dev
Then the following two files were used and compiled on the Jetson, and Raspberry:
Note: the files need to be compiled with the flag "-lbluetooth"
Example Server Code
int main(int argc, char **argv)
{
struct sockaddr_rc loc_addr = { 0 }, rem_addr = { 0 };
char buf[1024] = { 0 };
int s, client, bytes_read;
socklen_t opt = sizeof(rem_addr);
// allocate socket
s = socket(AF_BLUETOOTH, SOCK_STREAM, BTPROTO_RFCOMM);
// bind socket to port 1 of the first available
// local bluetooth adapter
loc_addr.rc_family = AF_BLUETOOTH;
loc_addr.rc_bdaddr = *BDADDR_ANY;
loc_addr.rc_channel = (uint8_t) 1;
bind(s, (struct sockaddr *)&loc_addr, sizeof(loc_addr));
// put socket into listening mode
listen(s, 1);
// accept one connection
client = accept(s, (struct sockaddr *)&rem_addr, &opt);
ba2str( &rem_addr.rc_bdaddr, buf );
fprintf(stderr, "accepted connection from %s\n", buf);
memset(buf, 0, sizeof(buf));
// read data from the client
while(true){ //continue running until error
bytes_read = read(client, buf, sizeof(buf));
if( bytes_read > 0 ) {
printf("received [%s]\n", buf);
//determine whether it was left or right turn
if(buf[0] == 'l'){
printf("LEFT SIGNAL...\n");
}
else if(buf[0] == 'r'){
printf("RIGHT SIGNAL...\n");
}
//otherwise throw error
}
}
// close connection
close(client);
close(s);
return 0;
}
Example Client Code
int main(int argc, char **argv)
{
struct sockaddr_rc addr = { 0 };
int s, status;
char dest[18] = "B8:27:EB:D8:59:7D"; //Bluetooth Address of device to connect to
char input; //reading input from keyboard
char buf[64];
// allocate a socket
s = socket(AF_BLUETOOTH, SOCK_STREAM, BTPROTO_RFCOMM);
// set the connection parameters (who to connect to)
addr.rc_family = AF_BLUETOOTH;
addr.rc_channel = (uint8_t) 1;
str2ba( dest, &addr.rc_bdaddr );
// connect to server
status = connect(s, (struct sockaddr *)&addr, sizeof(addr));
// send a message
if( status == 0 ) {
while(true){ //persistent data transfer
do{
scanf("%c", &input); //read from keyboard input
} while(input != 'l' | input != 'r');
buf[0] = input;
status = write(s, buf, 1); //send 1 char to server
}
}
if( status < 0 ) perror("error in sending data");
close(s);
return 0;
}